Hello and welcome!
Since last weeks blog, I have gone through a good chunk of the Bandit levels created by OverTheWire and in this blog I cherry picked some of the subjects that I learned about, including:
- Cron/crontab
- Brute-forcing
Cron
Cron is a daemon (a process that runs for long periods of time to service other requests) in which it has tasks scheduled at a specified time. In other words, if I wanted a particular script to execute at 3:00PM every other day that will backup files on the system, I can create a crontab script that will execute during that time. Cron will automate any tasks that the user has to perform at systematic time periods. In order to create a cron file, we must use the command crontab
. Some common commands with crontab
include:
-u
– specifies the user that you want the cron daemon to perform its automated commands – for ex. the user: root creates a crontab and specifies it to be used on another user: peter then we would saycrontab -u peter [file]
-l
– makes the crontab display on standard output-r
– removes the current crontab-e
– edit the current crontab- To read more about the commands of crontab:
crontab(1) — Linux manual page
In one of the Bandit levels I was required to read a crontab file and act based on the commands it had executed. The “time-string” is what dictates when the commands of the crontab will execute. There are 5 numeric placeholders that the programmer will specify – each number separated by a space character. The five placeholder numbers represent (starting from left to right):
- Position 1: This is the minute field where it is specified which minute on the clock we want it to execute (the values obviously range from 0-59 since it’s minutes)
- Position 2: This will be the hour field where we specify the hour of the day (this is in military time because the values range from 0-23)
- Position 3: You guessed it, this will specify the day of the month (with values ranging 1-31)
- Position 4: I bet you guessed it again – this is the month specified (values ranging from 1-12)
- Position 5: You specify here the day of the week when you want the command executed (values ranging from 0-7) where Sunday represents both 0 and 7
For example, if I wanted to execute a command to backup my system every Sunday at 1PM I would state the following: 0 12 * * 0
. Seems easy right? Oh you see the ‘*’ character in the sequence? This character states that you don’t specify a particular value in that sequence. Like in the example above, I didn’t care which month or day of the month for the command to be executed because I said I wanted it every Sunday. I learned crontab because a pre-made script was in one of the Bandit levels for me to solve. Imagine you have the power to automate commands at any given time. But wait, there’s more!
If we wanted a command to be executed, say every 15 minutes, we don’t care about the particular day, month, weekday, etc. To accomplish this we use the slash character (/) – let me show you how to accomplish the every 15 minute task:
*/15 * * * *
– what we are essentially doing here is we are making a condition for the time string to check. If the minute is divisible by 15 (hence we detect that it is the 15th minute mark) then it will execute the given command to that time string.
Example
Let’s say we want to automatically update Kali Linux (or any distro) using Cronjobs. As stated earlier we need to edit the crontab file by using the crontab -e command which will take us to the file to edit.
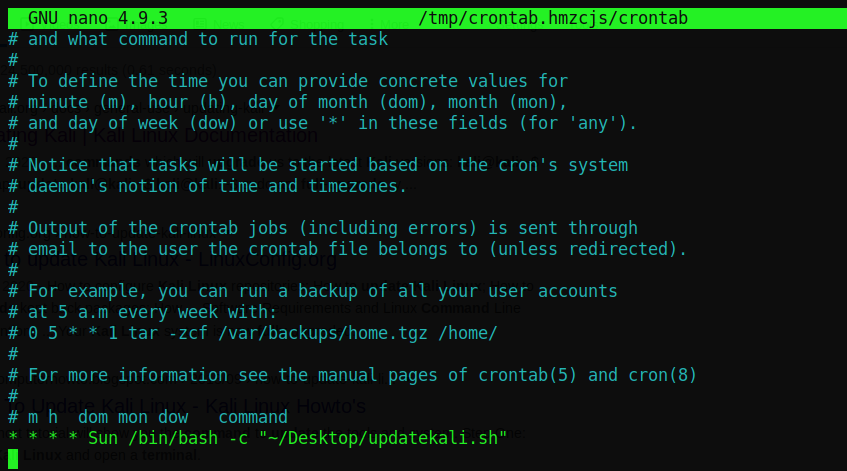
We see that there are hashtags for almost every line. This means that all the lines are commented out and the compiler will not run any of these lines – they will only run lines that do not have a # as the first character of the line. At the bottom of the file enter the following command that is in the picture: * * * * Sun /bin/bash -c “~/Desktop/updatekali.sh”. The command repeats every Sunday and we specify it to tell bash to run a script called updatekali.sh. Now make sure that you create the file in the path you specify or it will not work – in this case it is located on my Desktop. Now let’s create a file called updatekali.sh on the desktop. To do so we will cd to the desktop in our terminal and write the following command:
nano updatekali.sh
You will then be brought to a blank page where you will write the following bash script:
#!/bin/bash
apt update
apt full-upgrade
Save and close the file and that’s it! Your system will update every Sunday to make sure you have the latest tools! The idea of automating your commands at incremental or specific times is absolutely fascinating!
Brute-Forcing for Passwords
Brute-force is the action of repeatedly trying all variations of passwords as possible to login to a system of some sort. In one of the levels on OverTheWire they specify that you “may” need to brute-force your way to getting a password on a system. This particular level took me a good bit of time because I kept thinking that I had to use tools in Kali Linux to brute-force the password (which I didn’t). Here’s the thing though, that level was saying brute-force your way in but the way I completed it was more like a dictionary attack.
A dictionary attack is similar to brute-force but you actually use a dictionary of words that either you or someone else created to put as the password and you keep iterating through the dictionary until, hopefully, you come across the password. I say that it was a dictionary attack for the level because they gave me almost the whole password and stated “at the end of the password we gave you there are 4 digits after and you must find out which 4 digits is at the end of the password”. These are the steps I took to complete this level:
- I first thought to myself, since they gave me all this information of the password, I can just create a dictionary list (list of words you will use on the password credential) with every iteration of the password with 4 digits at the end of it (ex. password0001, password0002, password0003 until it gets to password9999). For this I used the tool crunch where it would help me make the dictionary list of passwords.
- Then I said to myself, “Now that I have a list of all possible passwords, how do I automate where I can execute the credentials every time?” Analyzing the system that I had to break into – there was a couple big flaws in it that allowed me to complete my attack:
- There is no limit of entering incorrect credentials so I can try as many times as I want and as fast as my computer can handle.
- I can create a bash script where I can automate entering the credentials until I get the correct password.
- Now that I analyzed the system and it’s flaws, it’s time to exploit it.
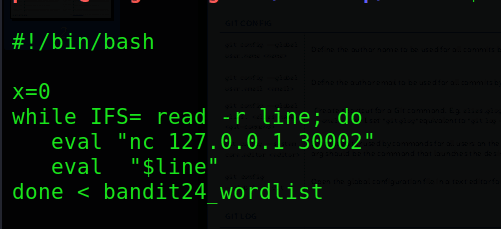
Honestly, and I’m sure you can see it, but this is not a very good script, and I am not showing it to show you my scripting skills, but just how I thought through the problem.
So, ignore the x=0
because that’s not supposed to be there, but lets start with the while IFS= read -r line; do
. In this line, we put the script in a while loop with the condition of “keep executing the code inside the while statement until we have reached the end of the file” and that file we are talking about is the dictionary list that we created earlier. We are also assigning the current line of text to the variable line
. Inside the ‘while’ block, we then use the eval
keyword (which tells bash to execute the following string that is specified). We execute the nc 127.0.0.1 30002
– which means we are connecting to the localhost on port 30002 with netcat.
Once we connect, we are then presented with a cursor that tells us to enter the password – where the next command eval "$line"
pastes the current line of the file (remember we assigned the line
variable the current line of text that the while loop was reading into from the dictionary list) into the password field. We also specified the file that we read in for the password at the end of the while statement with the line: done < bandit24_wordlist
. We then chmod +x
on the script to make it executable and we run it. It will iterate line after line of the dictionary list until the actual password is given – thus giving us the password to the next level.
Conclusion
I will definitely say this was a dense blog to say the least, but packed with lots of information. As I progress through these levels I see that hands-on experience seems to serve me the best in my studies for many reasons:
- It allows me to experience frustration which forces me to research more deeply on topics that I do not understand.
- Reinforces the knowledge I learn through the practice I put myself in.
- Makes me think about different ways I can solve a problem and when one way doesn’t work then I have to try to find another.
- So much more fun than just learning in lectures!
When presented a problem there are many things you have to consider – How can I solve it? Why didn’t the solution I use work? What other avenues can I go through to solve it? What were my mistakes on this level – what took me longer than usual to complete it? These are extremely important questions you have to keep asking yourself in order to find flaws in your methods and fix them. Remember, you cannot improve unless you work on your weaknesses and you are only as strong as your weakest link.
Take care and I will catch you all next week!
Peter